|
Example 1: Test gripper arm
The servomotor moves both arms of the gripper arm simultaneously. With the command setServo("S1", 60)
to the open position, with setServo("S1", 95)
the robot can grab objects. You may still need to adjust the angles. |
|
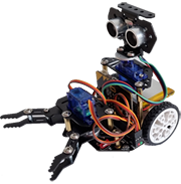
|
|
|
from mbrobot import *
#from mbrobot_plus import *
setServo("S1", 95)
delay(1000)
setServo("S1", 60)
delay(1000)
setServo("S1", 95)
► Copy to clipboard
Example 2: Grasping an object
The robot moves to the object located a short distance in front of it, grabs it with its gripper arm, moves back a short distance, turns to the left and places the object in the new location (similar to the video above). |
|
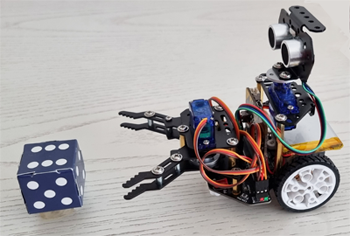
|
|
from mbrobot import *
#from mbrobot_plus import *
setServo("S1", 95)
delay(1000)
setServo("S1", 60)
forward()
delay(1000)
stop()
setServo("S1", 95)
backward()
delay(500)
left()
delay(700)
stop()
setServo("S1", 60)
► Copy to clipboard
Example 3: Positioning the gripper arm with an ultrasonic sensor
In example 2, the robot always starts at the same distance from the object and the length of the forward movement is determined by a time specification (delay(1000)). With the help of an ultrasonic sensor, the robot can recognise the correct position itself. The robot moves forwards until its distance to the object or to a wall behind it is less than 20 cm. It then grabs the object with its gripper arm and transports it away (similar to the video below).
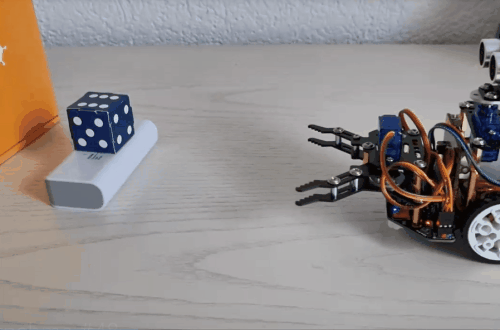
|
from mbrobot import *
#from mbrobot_plus import *
setServo("S1", 60)
forward()
repeat:
d = getDistance()
print(d)
if d < 20:
stop()
delay(500)
setServo("S1", 95)
delay(500)
backward()
delay(2500)
left()
delay(700)
stop()
setServo("S1", 60)
delay(100)
► Copy to clipboard
The distance to the wall is scanned every 100 milliseconds in an ‘endless’ repeat loop. If it is less than 20 cm, the robot stops and grabs the object with its gripper arm.
Example 4: Turn ultrasonic sensor with servomotor
The ultrasonic sensor is attached to a second servomotor in accordance with the assembly instructions above. This is connected to port S2. You can use the rotating ultrasonic sensor to search for objects (Exercise 3) or to have the robot follow a wall at a certain distance (Exercise 4).
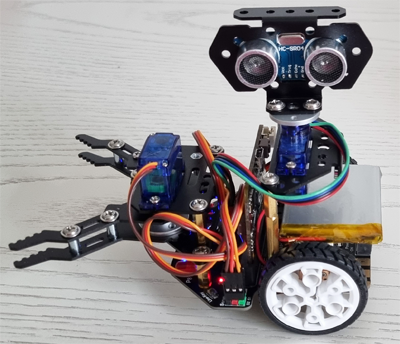
|
from mbrobot import *
#from mbrobot_plus import *
setServo("S2", 90)
delay(2000)
setServo("S2", 0)
delay(2000)
setServo("S2", 90)
delay(2000)
angle = 90
while angle >= 0:
setServo("S2", angle)
delay(500)
angle = angle - 10
angle = 0
while angle <= 90:
setServo("S2", angle)
delay(500)
angle = angle + 10
► Copy to clipboard
The programme shows how to turn the ultrasonic sensor with a second servomotor from the frontal position to a position that is parallel to the direction of travel. First in one step and then in small steps.
|